DKRZ NCL ICON (unstructured grid): draw vectors#
Example script:
;--------------------------------------------------------------------
; DKRZ NCL Example: draw_vectors_ICON.ncl
;
; Description: draw vectors (unstructured grid) using
; the weather symbols package WMAP
;
; 09.07.18 kmf
;--------------------------------------------------------------------
begin
r2d = get_r2d("float") ;-- radians to degrees
fu = addfile("U_10M_nwp_R2B09_lkm1006_atm3_2d_ml_20160801T000000Z.nc","r")
fv = addfile("V_10M_nwp_R2B09_lkm1006_atm3_2d_ml_20160801T000000Z.nc","r")
u = fu->U_10M(0,0,:) ;-- U-Component of wind
v = fv->V_10M(0,0,:) ;-- V-Component of wind
clon = tofloat(fu->clon * r2d) ;-- must be of type float
clat = tofloat(fu->clat * r2d) ;-- must be of type float
;-- define sub-region
lonmin = 0.0
lonmax = 120.0
latmin = 0.0
latmax = 60.0
;-- retrieve the indices of the cells within the sub-region; subset clat, clon, u and v
tmp_ind = ind((clon .ge. lonmin .and. clon .le. lonmax) .and. (clat .ge. latmin .and. clat .le. latmax))
clon_new = clon(tmp_ind)
clat_new = clat(tmp_ind)
u_new = u(tmp_ind)
v_new = v(tmp_ind)
;-- open workstation
wks_type = "png"
wks_type@wkWidth = 1200
wks_type@wkHeight = 1200
wks = gsn_open_wks(wks_type,"plot_draw_vectors_ICON")
;-- map resource settings
mpres = True
mpres@gsnFrame = False ;-- don't advance the frame
mpres@gsnMaximize = True ;-- maximize plot output
mpres@gsnLeftString = "Zonal Wind" ;-- left string
mpres@gsnRightString = "m/s" ;-- right string
mpres@mpOutlineOn = True ;-- outline map
mpres@mpMinLatF = latmin ;-- min lat
mpres@mpMaxLatF = latmax ;-- max lat
mpres@mpMinLonF = lonmin ;-- min lon
mpres@mpMaxLonF = lonmax ;-- max lon
mpres@tiMainString = "Unstructured grid (ICON): draw vectors~C~~Z80~workaround using WMAP package"
mpres@tiMainOffsetXF = -0.13 ;-- move title string to the left
mpres@tiMainOffsetYF = 0.04 ;-- move title string upward
map = gsn_csm_map(wks,mpres) ;-- create the map
;-- weather symbols settings for vectors
wmsetp("vrs - reference vector size",10.)
wmsetp("vrn - NDC size corresponding to vrs",0.03)
wmsetp("vcw - vector line width scale",2.0)
wmsetp("vch - vector head",0.005) ;-- black
wmsetp("vcc - vector color",1) ;-- black
inc = 1000 ;-- draw every inc'th vector (total number of vectors: ~21 million)
wmvectmap(wks, clat_new(::inc),clon_new(::inc),u_new(::inc),v_new(::inc)) ;-- draw the weather symbols: arrow
;-- draw label box
getvalues map ;-- retrieve the width, height, x- and y-position of the map
"vpWidthF" : vpw
"vpHeightF" : vph
"vpXF" : vpx
"vpYF" : vpy
end getvalues
wmvlbl(wks,(vpx+vpw),(vpy-vph)) ;-- draw label box (reference vector); x,y values: right lower corner
;-- advance the frame
frame(wks)
end
Result:
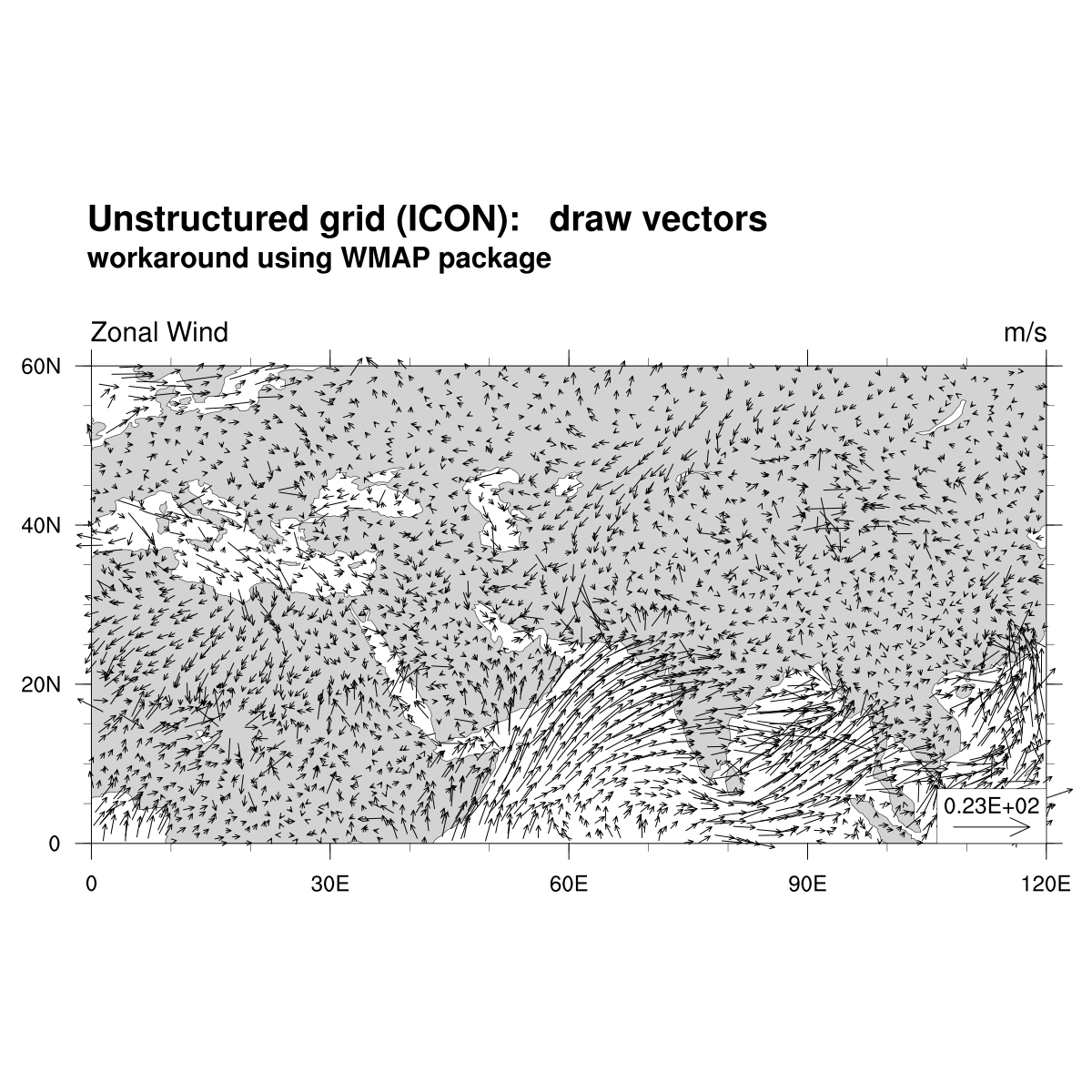